Implementing Secure Authorization in ABAP: Best Practices for Using AUTHORITY-CHECK
- Yasin Serinyel
- Mar 22
- 4 min read
Security in SAP systems is a critical aspect to ensure that users can only perform the actions they are authorized for. Improper authorization handling can lead to unauthorized data access, compliance violations, and security breaches. One of the fundamental mechanisms for authorization checks in ABAP is the AUTHORITY-CHECK statement. In this article, we will explore how AUTHORITY-CHECK works, common pitfalls, and best practices for its implementation. What is AUTHORITY-CHECK? AUTHORITY-CHECK is an ABAP statement used to verify whether a user has the required authorization. It works based on SAP authorization objects and prevents users from executing unauthorized actions. This mechanism is essential for enforcing security policies in custom developments and protecting sensitive business data.
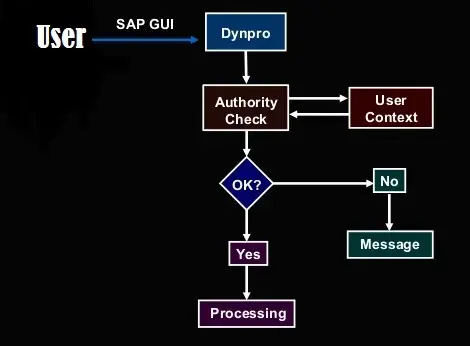
Using AUTHORITY-CHECK
To check if a user has a specific authorization, the AUTHORITY-CHECK OBJECT statement is used. The general syntax is as follows:
AUTHORITY-CHECK OBJECT 'ZYS_MY_AUTH'
ID 'ACTVT' FIELD '03'
ID 'COMPANY' FIELD 'TR01'.
IF sy-subrc <> 0.
MESSAGE 'You do not have permission!' TYPE 'E'.
ENDIF.
'Z_MY_AUTH': Authorization object that groups related security permissions.
'ACTVT' FIELD '03': Checks whether the user has permission for a specific action (03 = Read permission).
'COMPANY' FIELD 'TR01': Additional filtering within the authorization object, such as company code.
Common Mistakes to Avoid
1. Skipping Authorization Checks in Custom Developments
Custom ABAP developments should always include appropriate authorization checks. Assuming that SAP standard role assignments are sufficient can lead to unauthorized access. 2. Hardcoding Authorization Objects
Avoid hardcoding authorization values in the program. Instead, use configurable values or retrieve them dynamically.
Incorrect:
AUTHORITY-CHECK OBJECT 'ZYS_MY_AUTH' ID 'ACTVT' FIELD '03'.
Better approach:
data(lv_act) = '03'.
AUTHORITY-CHECK OBJECT 'ZYS_MY_AUTH' ID 'ACTVT' FIELD lv_act.
Best Practices for Secure Implementation
To use authorization checks effectively and securely, consider the following best practices:
1. Define Authorization Objects Properly
For custom authorization checks, create custom authorization objects starting with Z*. If standard SAP authorization objects are insufficient, new ones can be defined using transaction SU21.
2. Centralize AUTHORITY-CHECK Usage
Instead of using AUTHORITY-CHECK repeatedly in different parts of the code, centralizing the check in a Function Module (FM) or Class Method improves maintainability and reduces code duplication.
Example:
CLASS zcl_auth CHECK FINAL.
CLASS-METHODS check_user_auth IMPORTING iv_act TYPE char2 RETURNING VALUE(rv_auth) TYPE abap_bool.
ENDCLASS.
METHOD zcl_auth=>check_user_auth.
AUTHORITY-CHECK OBJECT 'ZYS_MY_AUTH' ID 'ACTVT' FIELD iv_act.
rv_auth = xsdbool( sy-subrc = 0 ).
ENDMETHOD.
Usage:
IF zcl_auth=>check_user_auth('03') = abap_false.
MESSAGE 'You do not have permission!' TYPE 'E'.
ENDIF.
3. Leverage SAP Standard Authorization Mechanisms
SAP supports the creation of authorization profiles via PFCG (Profile Generator). Assigning authorizations through user roles minimizes the need for extensive authorization checks within the code.
4. Log Authorization Checks
Logging unauthorized access attempts enhances system security. Authorization checks can be monitored using transaction ST01.
Example logging:
DATA: lv_activity TYPE string,
lv_company TYPE string,
lv_timestamp TYPE string,
lv_user TYPE sy-uname,
lv_message TYPE string.
lv_activity = '03'. " Activity: Display
lv_company = 'TR01'. " Company: TR01
lv_timestamp = sy-datum && ' ' && sy-uzeit.
" Perform AUTHORITY-CHECK
AUTHORITY-CHECK OBJECT 'ZYS_MY_AUTH'
ID 'ACTVT' FIELD lv_activity
ID 'COMPANY' FIELD lv_company.
IF sy-subrc <> 0.
" Log the unauthorized access attempt
lv_user = sy-uname.
lv_message = |Unauthorized access attempt by user { lv_user } for Object 'ZYS_MY_AUTH' at { lv_timestamp } |.
" Write the log entry to a custom table or file for auditing
INSERT VALUE #( uname = lv_user
timestamp = lv_timestamp
object = 'ZYS_MY_AUTH'
activity = lv_activity
company = lv_company
message = lv_message ) INTO zys_authlog_table.
" Optionally, send email alert or log to external monitoring system
CALL FUNCTION 'SO_NEW_DOCUMENT_SEND_API1'
EXPORTING
document_data = lv_message
recipients = 'it.security.chief@company.com'
EXCEPTIONS
others = 1.
" Display a generic error message to the user
MESSAGE 'You do not have permission to perform this action.' TYPE 'E'.
ENDIF.
5. Ensure User-Friendly Error Handling
Avoid terminating the program abruptly due to authorization failures. Instead, provide user-friendly messages and guide users on obtaining the necessary permissions.
Incorrect usage:
AUTHORITY-CHECK OBJECT 'ZYS_MY_AUTH'.
IF sy-subrc <> 0.
EXIT. " Immediately terminates the program.
ENDIF.
Correct usage:
IF sy-subrc <> 0.
MESSAGE 'You are not authorized to perform this action! Please contact your administrator.' TYPE 'E'.
ENDIF.
Performance Considerations
While authorization checks are crucial for security, excessive or redundant checks can impact performance. To optimize performance:
Place AUTHORITY-CHECK statements at the beginning of transactions or function modules to verify user permissions early, avoiding repeated checks during data processing.
Minimize unnecessary checks within loops or for each data entry, as repetitive authorization checks can significantly degrade performance. Instead, check once per session or for a group of related actions.
Leverage authorization buffering mechanisms for frequently checked permissions. This reduces database load and enhances response times by keeping authorization data in memory for quicker access during multiple checks.
Conclusion
AUTHORITY-CHECK is a key mechanism for implementing authorization controls in SAP ABAP. Defining authorization objects correctly, centralizing authorization checks, leveraging SAP's standard mechanisms, and ensuring user-friendly error handling are essential for building a secure system. Additionally, avoiding common mistakes and optimizing performance can further enhance security and efficiency.
If you are interested in ABAP security, regularly reviewing your authorization processes and ensuring your code complies with SAP security standards is crucial.
Comentários